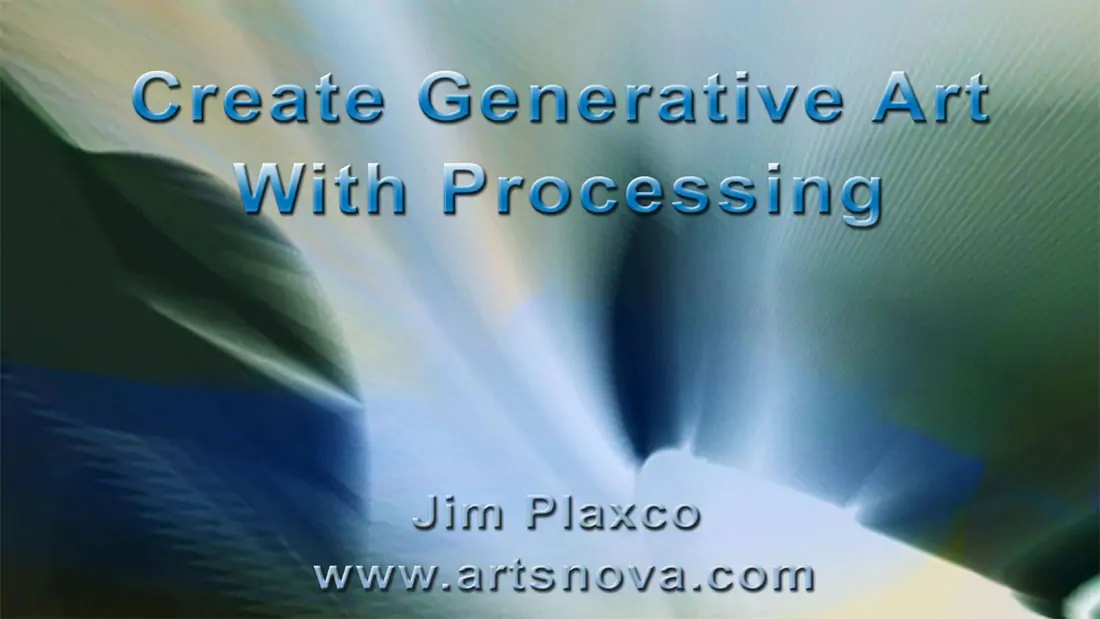
Create Generative Art With Processing Workshop for the 2022 Chicon World Science Fiction Convention
Processing is a programming language designed for use by artists in order to facilitate the creation of digital art, which includes generative art. Workshop participants will learn the essentials of the Processing programming language and will receive an introduction to a generative art and creative coding design philosophy. Each participant will leave having successfully created their own work of generative art. Participants must have Processing installed on their laptops prior to the start of the session. Processing for Linux, Macs, and Windows can be downloaded from processing.org/download
The main objectives for this workshop are to:
- address how to approach creative coding and the creation of generative art
- discuss a creative coding program design philosophy
- cover the basics of the Processing IDE (Integrated Development Environment) and the language basics
- create a working program and an original generative artwork.
Topical sections of the workshop presentation will address the following subjects:
- Answering the question of what is generative art?
- Describing various generative art creation concepts?
- Answering the question of what is creative coding?
- Developing a creative coding design philosophy
- Exploring the Processing IDE
- Introducing the Processing language
- As a class project: creating a 3D Generative Art Animation
The workshop will conclude with a question and answer session
To facilitate the learning process, the following two examples of Processing programs can used for experimenting with the Processing language, the Processing IDE, and the creative coding process.
Example Processing Program 1: A Simple Interactive Animated Circle
//---------------------------------------------------------------------------- // Sample program Number 1 // For Chicon 2022 Create Generative Art With 'Processing' workshop // By Jim Plaxco, www.artsnova.com //---------------------------------------------------------------------------- // GLOBAL VARIABLES String aString = "This gets printed to the console"; // A String variable int aCounter = 0; int ellipseX, ellipseY; // setup() is where your program initializes itself void setup() { size(800,800); // set the size of the canvas background(0); // make the canvas black fill(255); // fill shapes with white stroke(0); // draw lines with black strokeWeight(2); // make lines 2 pixels thick println(aString); // print the contents of the variable aString to the console window ellipseX=width/2; // set the variable ellipseX to 1/2 the canvas width ellipseY=height/2;// set the variable ellipseY to 1/2 the canvas height } // end of the setup() function // draw() runs in an infinite loop void draw() { ellipse(ellipseX,ellipseY, aCounter,aCounter); // draw an ellipse at x,y of size aCounter aCounter +=10; // increment the value of aCounter by 10 if(aCounter > width) aCounter = 10; // if the value of aCounter is greater than // the canvas width, set its value to 10 } // end of the draw() function // keyPressed() is run when a key on the keyboard is pressed void keyPressed(){ if(key == 's') { // if the key pressed was the 's' key saveFrame("chicon-test-output-####.png"); // save the current canvas as a PNG file println("Saved"); // write the string Saved to the console window } } // end of the keyPressed() function // mousePressed() is run when a mouse button (left or right) is pressed void mousePressed() { ellipseX=mouseX; // set the value of the variable ellipseX to the value of mouseX ellipseY=mouseY; // set the value of the variable ellipseY to the value of mouseY } // end of the mousePressed() function
Example Processing Program 1: An Implementation of Sol Lewitt's wall drawing of 50 dots
//---------------------------------------------------------------------------- // Implementation of Sol Lewitt algorithm wall drawing of 50 dots // For Chicon 2022 Create Generative Art With 'Processing' workshop // This program uses the Object Oriented Programming (OOP) concept of Classes // By Jim Plaxco, www.artsnova.com //---------------------------------------------------------------------------- // Global Variables int numDots = 50; int area, areaBlock; int segmentlength; DOTS[] theDots; // setup() function void setup() { size(1200,1200); background(255); strokeWeight(1); stroke(0); area = width * height; areaBlock = area / 50; segmentlength = int(sqrt(areaBlock)); println("segmentlength="+segmentlength); theDots = new DOTS[numDots]; int aindx = 0; for(int ix = 0; ix < width; ix += segmentlength) { for(int iy = 0; iy < height; iy += segmentlength) { int xv = int(random(ix, ix+segmentlength)); if (xv > width) xv = int(random(ix, width)); int yv = int(random(iy, iy+segmentlength)); if (yv > height) yv = int(random(iy,height)); if(aindx < theDots.length) theDots[aindx] = new DOTS(xv,yv); aindx++; } } } // draw() function void draw() { for(int s = 0; s < theDots.length; s++) { for(int d = s+1; d < theDots.length; d ++) { line(theDots[s].x, theDots[s].y, theDots[d].x, theDots[d].y); } } } // keyPressed() function void keyPressed(){ if(key == 's') { saveFrame("sol-lewitt-50-dots-####.png"); // save the current canvas as a PNG file println("Saved"); } } // DOTS class class DOTS { int x,y; DOTS(int xv, int yv) { x = xv; y = yv; } }
The two programs provided here are free for creative coders to use and experiment with in their pursuit of the creation of digital art. Enjoy.